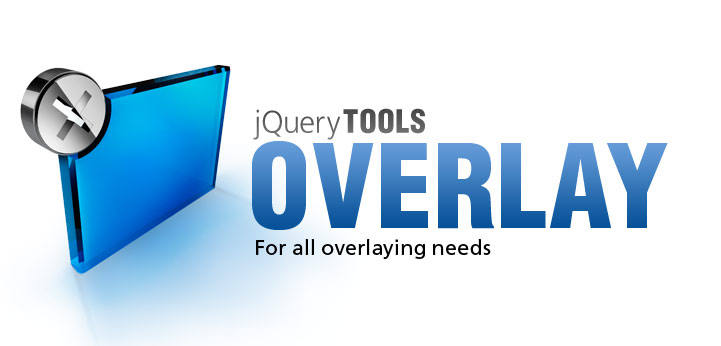
Creating modal dialogs with overlay
This demo shows you how you can use the overlay tool to prompt for user input. You may be familiar with the old school prompt() call in JavaScript. Now let's get into the modern world and use JavaScript more innovatively:
This is a modal dialog
You can only interact with elements that are inside this dialog. To close it click a button or use the ESC key.
This is a modal dialog
You can only interact with elements that are inside this dialog. To close it click a button or use the ESC key.
The "Modal dialog" is an overlay that requires the user to interact with it before they can return to using the main document. This feature can be achieved with the Expose tool which is tightly integrated with this overlay tool.
There are two major advantages over the default JavaScript prompts:
- You can tweak the look and feel of the dialog box
- You can ask any kind of questions and have multiple input fields.
HTML coding
Again, the HTML coding is no different from the basic setup. There are trigger elements and their corresponding overlay elements. Note that we have explicitly defined the close buttons here by assigning the class name "close" to the buttons. This will automatically bind the close actions to them.
<!-- the triggers -->
<p>
<button class="modalInput" rel="#yesno">Yes or no?</button>
<button class="modalInput" rel="#prompt">User input</button>
</p>
<!-- yes/no dialog -->
<div class="modal" id="yesno">
<h2>This is a modal dialog</h2>
<p>
You can only interact with elements that are inside this dialog.
To close it click a button or use the ESC key.
</p>
<!-- yes/no buttons -->
<p>
<button class="close"> Yes </button>
<button class="close"> No </button>
</p>
</div>
<!-- user input dialog -->
<div class="modal" id="prompt">
<h2>This is a modal dialog</h2>
<p>
You can only interact with elements that are inside this dialog.
To close it click a button or use the ESC key.
</p>
<!-- input form. you can press enter too -->
<form>
<input />
<button type="submit"> OK </button>
<button type="button" class="close"> Cancel </button>
</form>
<br />
</div>
CSS coding
Nothing special here. We style the dialog and a few elements inside it:
.modal {
background-color:#fff;
display:none;
width:350px;
padding:15px;
text-align:left;
border:2px solid #333;
opacity:0.8;
-moz-border-radius:6px;
-webkit-border-radius:6px;
-moz-box-shadow: 0 0 50px #ccc;
-webkit-box-shadow: 0 0 50px #ccc;
}
.modal h2 {
background:url(/media/img/global/info.png) 0 50% no-repeat;
margin:0px;
padding:10px 0 10px 45px;
border-bottom:1px solid #333;
font-size:20px;
}
JavaScript coding
First we enable those trigger elements to open our dialogs. We select the elements with the jQuery selector .modalInput and initialize overlaying with two configuration variables. The mask variable enables the semitransparent mask and we give it the color "#ebecff". By setting closeOnClick variable to false we enable our "modal" feature: users cannot interact with the main document when the overlay is open because the rest of the document is disabled by the mask until the dialog is closed.
var triggers = $(".modalInput").overlay({
// some mask tweaks suitable for modal dialogs
mask: {
color: '#ebecff',
loadSpeed: 200,
opacity: 0.9
},
closeOnClick: false
});
Yes/No prompt
Here is the code for the yes/no prompt. We bind a click event to both buttons and we know which one of the buttons is clicked by using jQuery's index method. After we know the result we simply write the answer to the trigger. Of course you should do something more useful with the result.
var buttons = $("#yesno button").click(function(e) {
// get user input
var yes = buttons.index(this) === 0;
// do something with the answer
triggers.eq(0).html("You clicked " + (yes ? "yes" : "no"));
});
Input prompt
Here we bind a submit event handler for the FORM element inside the dialog. This handler closes the overlay by using the overlay API call close. The default form submission action can be cancelled by returning e.preventDefault().
$("#prompt form").submit(function(e) {
// close the overlay
triggers.eq(1).overlay().close();
// get user input
var input = $("input", this).val();
// do something with the answer
triggers.eq(1).html(input);
// do not submit the form
return e.preventDefault();
});