
Custom tooltip effect
Here you can see a customized "bouncy" tooltip effect. We set up things similarly as in the basic setup, but we have modified the sliding effect with our own:
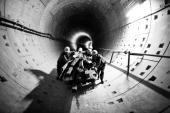
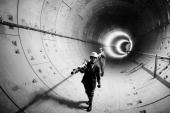
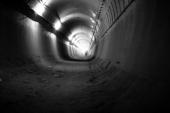
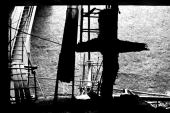
HTML coding
A simple setup with the tooltip element and the triggers:
<!-- tooltip triggers -->
<img src="image1.jpg" title="The tooltip text #1"/>
<img src="image2.jpg" title="The tooltip text #2"/>
<img src="image3.jpg" title="The tooltip text #3"/>
<img src="image4.jpg" title="The tooltip text #4"/>
Creating the effect
We start by adding a new animation algorithm for jQuery called "bouncy". This is possible by extending the jQuery.easing object. I grabbed this easing function from the jQuery Easing Plugin's source code. There are quite a lot of different easing algorithms that you can try.
Custom tooltip effects are done with the $.tools.tooltip.addEffect method. The first argument is the effect name, the second argument is the function that defines how the tooltip is shown and the third argument defines how the tooltip closes. Inside the functions the this variable is a reference to the tooltip API.
// create custom animation algorithm for jQuery called "bouncy"
$.easing.bouncy = function (x, t, b, c, d) {
var s = 1.70158;
if ((t/=d/2) < 1) return c/2*(t*t*(((s*=(1.525))+1)*t - s)) + b;
return c/2*((t-=2)*t*(((s*=(1.525))+1)*t + s) + 2) + b;
}
// create custom tooltip effect for jQuery Tooltip
$.tools.tooltip.addEffect(
"bouncy",
// opening animation
function(done) {
this.getTip().animate({top: '+=15'}, 500, 'bouncy', done).show();
},
// closing animation
function(done) {
this.getTip().animate({top: '-=15'}, 500, 'bouncy', function() {
$(this).hide();
done.call();
});
}
);
Note: you can get access to the configuration option with the this.getConf() method, and you can use this object to supply custom configuration options as well.
JavaScript coding
Here is the tooltip configuration. We supply our newly created tooltip effect with the effect configuration variable.
$("#demo img[title]").tooltip({effect: 'bouncy'});